Synthetic monitoring as Code with Checkly and ilert
This post will introduce Checkly, the synthetic monitoring solution, and their monitoring as code approach. This guest post was written by Hannes Lenke, the CEO, and co-founder of Checkly.
First, thanks to Birol and the ilert team for the opportunity to introduce Checkly. ilert recently announced discontinuing its uptime monitoring feature and worked with us on an integration to ensure that existing customers could migrate seamlessly.
So, what is monitoring as code and Checkly? Let’s dive in!
Checkly and monitoring as code via Terraform and CLI
Checkly is the synthetic monitoring solution for modern Developers, DevOps, SRE, and Platform Engineering teams with hundreds of customers worldwide using the platform to ship software confidently. It allows configuring globally distributed API and browser monitors that trigger alerts when things fail.
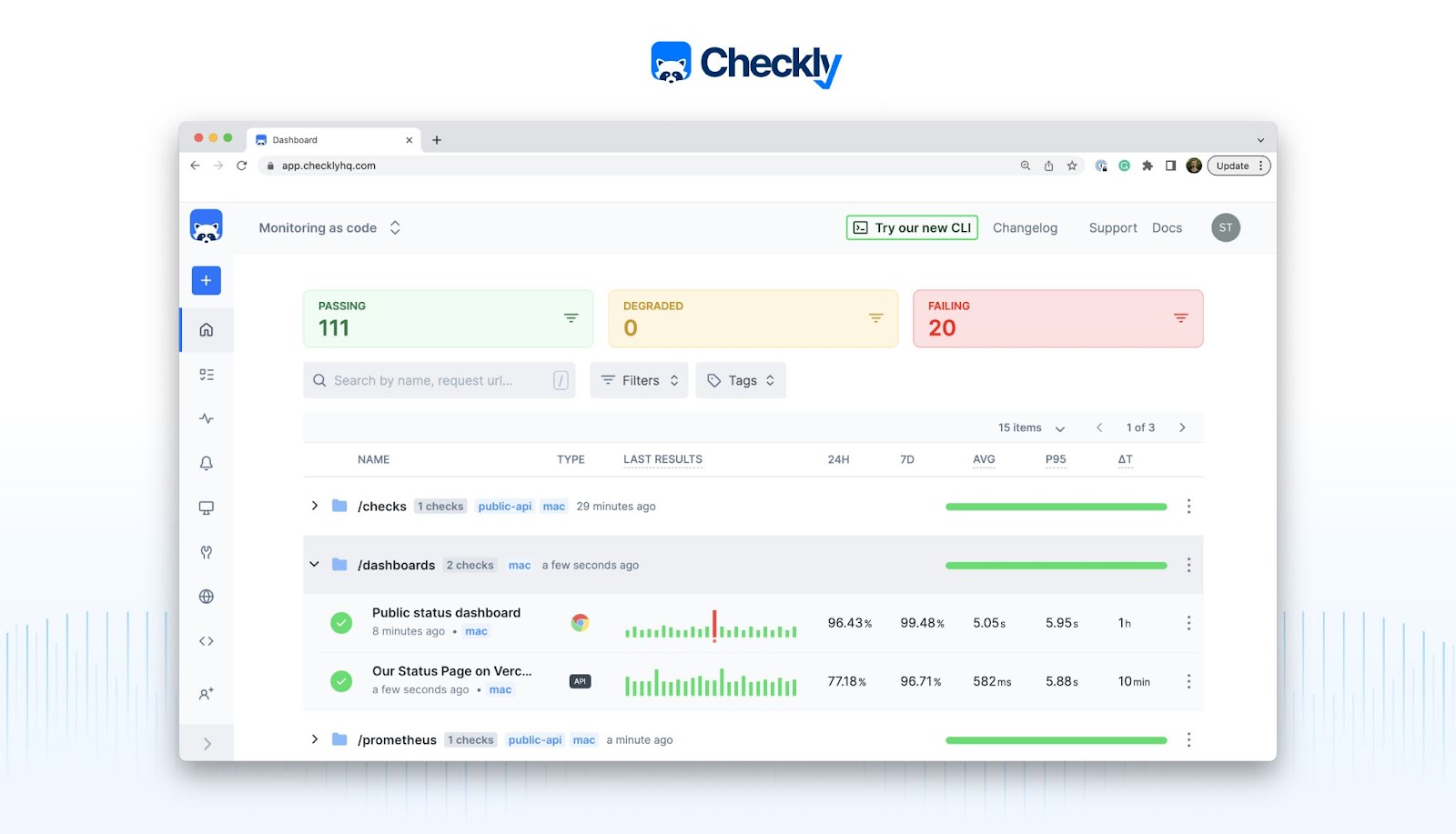
At Checkly, we believe monitoring should start as code, be automated, and live in your repository. Monitoring as code allows you to code, test, and deploy API and browser checks from your code repository and run these as tests or scheduled as monitors.
Currently, Checkly supports three tools for the monitoring as code workflow:
- The Checkly CLI
- A Terraform provider
- A Pulumi provider
The best tool depends on your goals and how your organization is set up.
Let’s dive in and learn how to set up monitors via Terraform or the Checkly CLI.
Configuring monitors via Terraform
The Checkly Terraform provider lets you declare your setup using HashiCorp Terraform. Start with it by following the steps in the getting started guide.
But in summary: add the Checkly Terraform provider to your project, set your credentials, and configure API and Browser monitors via the common Terraform syntax.
For example, add an API check resource at the bottom of your existing terraform file to create an API monitor.
This API check monitors api.example.com once a minute, runs in two locations (us-west-1 and eu-central-1), and tests for a proper 200 status code.
But your monitoring stack shouldn’t only consist of API checks!
Headless browser monitoring enables you to test your apps’ end-user experience continuously. A headless browser check can be configured via the following Terraform code:
The defined browser check uses Playwright (a modern JavaScript testing framework) to spin up a browser and test if the site at playwright.dev is delivered with the correct title. The check runs every five minutes in two locations (eu-central-1 and us-west-1) and the double_check parameter defines that it will be retried in case of failure to ensure reliability.
Now that you defined your checks, you’ll want to set up an alert channel to be promptly informed when one fails. Follow this guide, create a new Checkly alert source in ilert, and feed it with monitoring failures from Checkly.
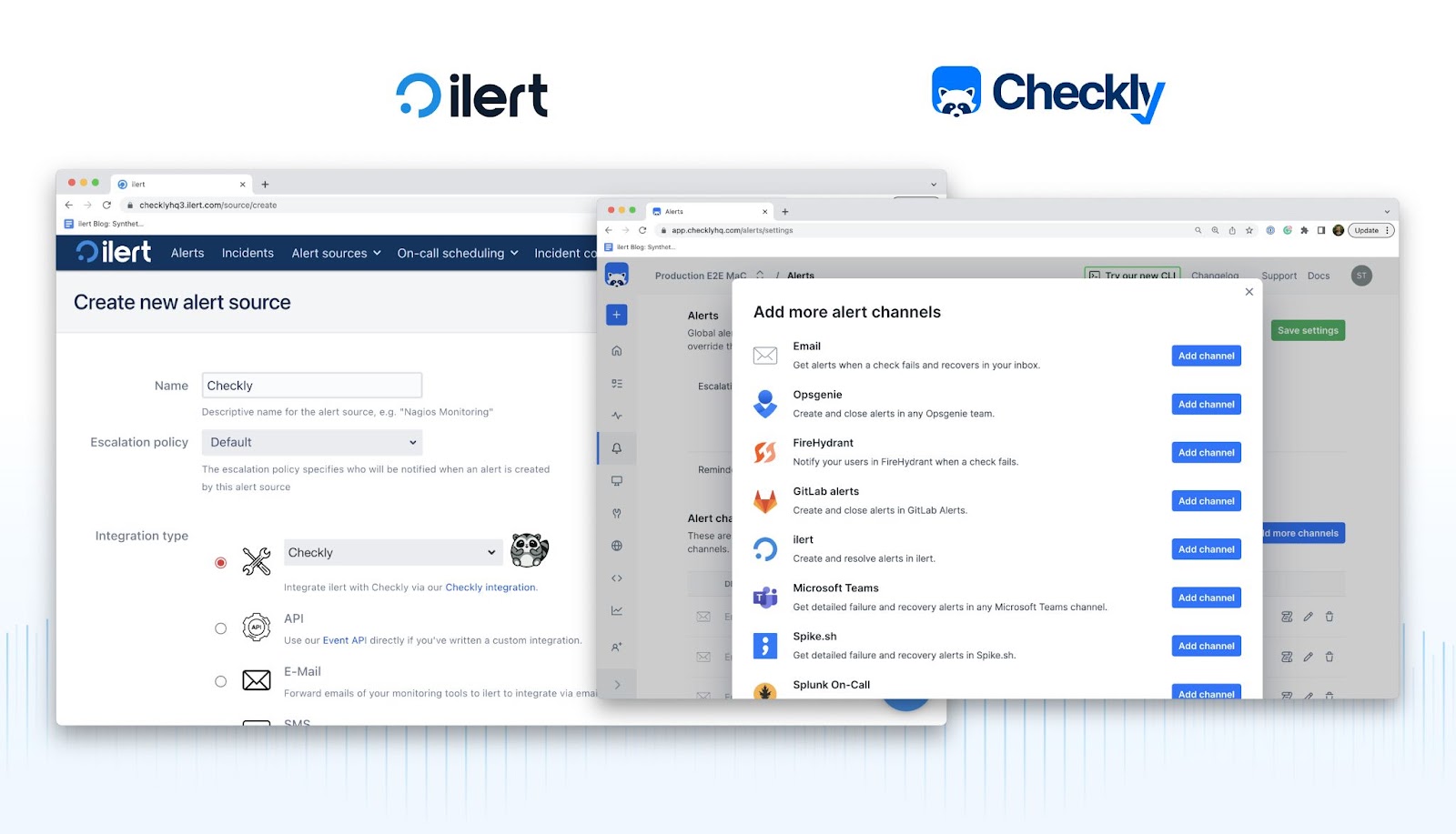
Connecting both services takes only a couple of minutes!
But what if you aren’t keen on using Terraform?
Configuring monitors via the Checkly CLI
The Checkly CLI provides a JavaScript/TypeScript-native workflow from your code base for coding, testing, and deploying synthetic monitoring at scale. It comes with native Playwright Test support, and similar to the Terraform provider, it allows you to provision your monitoring infrastructure in code.
The primary advantages of the Checkly CLI are:
- It unites end-to-end testing & monitoring in one workflow.
- It’s programmable, testable, and reviewable.
- It works with your development pipeline. From your IDE, via CI to production.
- It supports Playwright Test natively. (No lock-in; just write standard *.spec.ts files).
- It allows you to define and code your alert channel resources. Set alerts for Slack, SMS, and many more channels.
- It’s Typescript-first and fully typed for a stellar developer experience with code completion.
- It can run in the cloud or your network using Private Locations.
The recommended way to bootstrap a new Checkly CLI project is to run the following command.
Follow our docs, if you want to get started and are looking for detailed setup instructions.
While there are many details about monitoring as code with the Checkly CLI, this article highlights the basic concepts for creating API and browser checks.
Create a basic API check pinging the public Checkly API in an api-check.check.ts file as follows.
Define browser checks via plain Playwright Test syntax and import these automatically from your repository via the global check match configuration.
Below is a browser check example of logging into a web store to test the login routine.
Similarly to the Terraform Provider, the CLI allows us to set up alerts to ensure we are informed as soon as a failure occurs. Alert channels can be declared as constructs, just like the API and browser checks, you’ve already seen above.
Let’s add the following to an alert-channels.ts file in our Checkly CLI project:
This code defines an alert channel but it isn’t enough to get alerted about failed checks. Why not?
To receive alerts, you must programmatically connect checks with alert channels via the alertChannels property. This way, you’re in full control of your checks and the alerts they trigger.
After defining all the resources, a quick `checkly deploy` will apply the changes to our Checkly account. We’re now fully up, and our checks will run on schedule, informing us promptly if anything was to go wrong. Rapidly learning about failures in your API and essential website flows allows us to respond swiftly and minimize any negative impact on your users.
Sum up
If you're an ilert user using Terraform, great! You'll be pleased to know that Checkly is fully compatible with this workflow and integrates seamlessly with ilert, making it an excellent option for those looking for a new uptime monitoring service.
If you’re not using an infrastructure as code solution but still want to adopt monitoring as code, the Checkly CLI is the perfect solution to get you started.
And in any case, If you're interested in learning more about Checkly, we'd be happy to provide a demo and invite you to test it out at checklyhq.com.